Table Of Content

Continue your learning by reading about other practices for Agile and Adaptive software development. This code establishes that both the high-level and low-level modules depend on abstraction. First, the MySQLConnection is the low-level module while the PasswordReminder is high level, but according to the definition of D in SOLID, which states to Depend on abstraction, not on concretions.
Modeling languages
This makes your software easier to read, understand, and modify, leading to more robust and maintainable applications. Encapsulation – Encapsulation is one of the fundamental concepts in object-oriented programming (OOP). It describes the idea of wrapping data and the methods that work on data within one unit, e.g., a class in Java.
How Do You Inherit From Another Class in Python?
Every time you create a new Dog object, .__init__() sets the initial state of the object by assigning the values of the object’s properties. This principle is the first principle that applies to Interfaces instead of classes in SOLID and it is similar to the single responsibility principle. It states that clients should not be forced to depend upon interface members they do not use. This means that the interface should have the minimal set of methods required to ensure functionality and be limited to only one functionality.
Liskov Substitution Principle (LSP)
For example, when you click on an icon to open an application, you don't need to know how the laptop's processor retrieves and executes the application's code. You simply interact with the interface at a high level of abstraction, without needing to worry about the low-level details. Developers create public methods that allow others to call methods on an object, ideally with accompanying documentation.
Object-Oriented Programming (OOP) in Python 3
In this article we will go over Object Oriented Programming (OOP) as a whole, without relying on a particular language. You'll learn what it is, why it's so popular as a programming paradigm, its structure, how it works, its principles, and more. Although most programming languages have some similarities, each one has specific rules and methods which makes it unique. Mastering the concepts of object-oriented programming is essential for any software developer looking to create efficient, maintainable, and scalable software systems. By understanding these core concepts, you will be well on your way to leveraging the full potential of OOP in your projects. The useExtraAbility() method is defined in the child SpecialIronMan class, so objects created from the SpecialIronMan class, like a suit with invisibility, have access to the useExtraAbility() method.
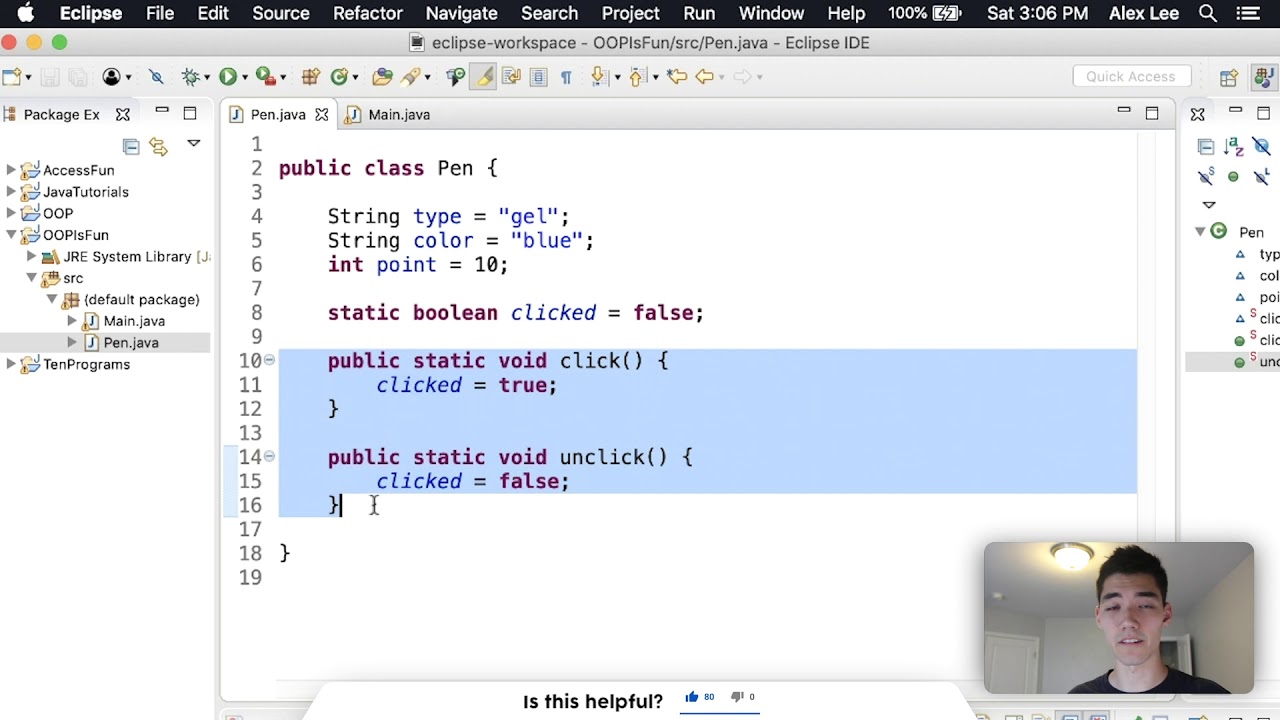

The Visitor Pattern used in situations where you have a set of objects with different types or classes and you want to perform a common operation on all of them. The Observer Pattern is a behavioral desing pattern in object-oriented programming. It defiens a one-to-many dependency between objects so that when one object (the subject) changes its state, all its dependents (observers) are notified and updated automatically.
This snippet above violates this principle as the PasswordReminder class is being forced to depend on the MySQLConnection class. Now in AreaCalculator class, you can replace the call to the area method with calculate and also check if the object is an instance of the ManageShapeInterface and not the ShapeInterface. A client should never be forced to implement an interface that it doesn’t use, or clients shouldn’t be forced to depend on methods they do not use. This means that every subclass or derived class should be substitutable for their base or parent class. The SumCalculatorOutputter class handles the logic needed to output the data to the user.
History and Evolution of OOP
This is often because they will be used for various programming methods. The program will determine which usage is critical for every execution of the thing from the parent class which reduces code duplication. It also allows different kinds of objects to interact with the same interface. It took me some time to really understand it's importance in programming. But this also doubled as an opportunity for me to learn its key concepts, and know how important it is for a developer's career and being able to solve challenges. One concept that is common among many programming languages is Object Oriented Programming.
Design Patterns with Python for Machine Learning Engineers: Prototype - Towards Data Science
Design Patterns with Python for Machine Learning Engineers: Prototype.
Posted: Tue, 05 Dec 2023 08:00:00 GMT [source]
In OOP, we create classes that serve as blueprints for constructing objects. Objects are instances of these classes, and they interact with one another using methods that define their behavior. This structure emphasizes modularity, reusability, and maintainability, which I found to be incredibly helpful when working on larger, more intricate projects. However, the true potential of OOP wasn't fully realized until the 1980s, when the Smalltalk language emerged. With its innovative design, Smalltalk made OOP more accessible and versatile, leading to its widespread adoption. Since that pivotal moment, numerous programming languages have embraced OOP principles, including Java, C++, Python, and C#.
Tips on writing code for Data-Oriented Design - Game Developer
Tips on writing code for Data-Oriented Design.
Posted: Tue, 09 Apr 2019 07:00:00 GMT [source]
You start all class definitions with the class keyword, then add the name of the class and a colon. Python will consider any code that you indent below the class definition as part of the class’s body. A great way to make this type of code more manageable and more maintainable is to use classes. The book uses Python’s built-in IDLE editor to create and edit Python files and interact with the Python shell, so you’ll see occasional references to IDLE throughout this tutorial.
Inheritance can be performed by cloning the maps (sometimes called "prototyping"). Object-oriented design (OOD) is the process of planning a system of interacting objects to solve a software problem. By defining classes and their functionality for their children (instantiated objects), each object can run the same implementation of the class with its state. The Dog class inherits the properties and behaviors of Animal class and can also have its own additional members. This code structure promotes code reuse and the modeling of relationships between different classes in a hierarchical manner.
Coupling is the degree of connectivity among things, that is how your piece of code is connected to each other. Simply, if your code is talking about another piece of code, there is coupling. Now as per this principle, either remove or minimize dependency to the extent it could be.
The most common is known as the design patterns codified by Gamma et al.. More broadly, the term "design patterns" can be used to refer to any general, repeatable, solution pattern to a commonly occurring problem in software design. Some of these commonly occurring problems have implications and solutions particular to object-oriented development. The Document Object Model of HTML, XHTML, and XML documents on the Internet has bindings to the popular JavaScript/ECMAScript language. JavaScript is perhaps the best known prototype-based programming language, which employs cloning from prototypes rather than inheriting from a class (contrast to class-based programming). Here, the class car has properties car_id, colour, engine_no, and distance.
Inheritance is a mechanism that allows a class to inherit the attributes and methods of another class. In simple terms, child classes get attributes and behaviors from parent classes. Mastering object-oriented programming requires understanding the fundamental principles and best practices, as well as how and when to use common design patterns. Remember, the ultimate goal of mastering OOP is to write code that not only works, but is also clean and manageable.
It resembles the real-world car that has the same specifications, which can be declared public (visible to everyone outside the class), protected, and private (visible to none). Also, there are some methods such as distance_travelled(), petrol_used(), music_player() and display(). In the code given below, the car is a class and c1 is an object of the car.
No comments:
Post a Comment